Image control
SCADE 2.0 - Displaying images using Swift on Android and iOS
Source code
Please see the UgBitmapDemo project for the source code created in this chapter. See Installing examples for information on how to download the examples. The URL is here for direct access is <https://github.com/scadedoc/UgExamples/tree/master/UgBitmapDemo2>
Introduction
Using the bitmap control, you can display images sourced from within the app or dynamically downloaded from the internet.
As with all of our controls, the controls represent a wrapper around the native controls and expose a subset of their functionality to users:
- We support the following formats : PNG, SVG, and JPG
- We support SVG-based scaling of the bitmap
Image Control attributes
Attribute Name | Attribute Type | Attribute Meaning | Example |
---|---|---|---|
content | String! | Field to store the actual image data that you want to display | Binary data |
url | String! | Relative path to the source of the image. Make sure you specify the correct path. It starts with the name of the resource folder, followed by the image name. You can only specify relative URLs that are local to the app directory structure. External URLs such as http:\...\myimage.png are currently not supported. | Correct res/dog.png Wrong: /Appname/res/dog.png /res/dog/png * http:\...\mydog.png |
isContentPriority | Bool | Defines which inbound source is used to display the image False, i.e. image data is retrieved using information in url field True, i.e. image data is taken from the content field |
Static image references
The image control serves the purpose of adding new images to an app, but it isn't active until you've placed one or more images/bitmaps in the Assets folder (or a subfolder).
Use the IMAGE Path found on the lower end of the Page Editor Panel to set a bitmap on the image control you have selected:
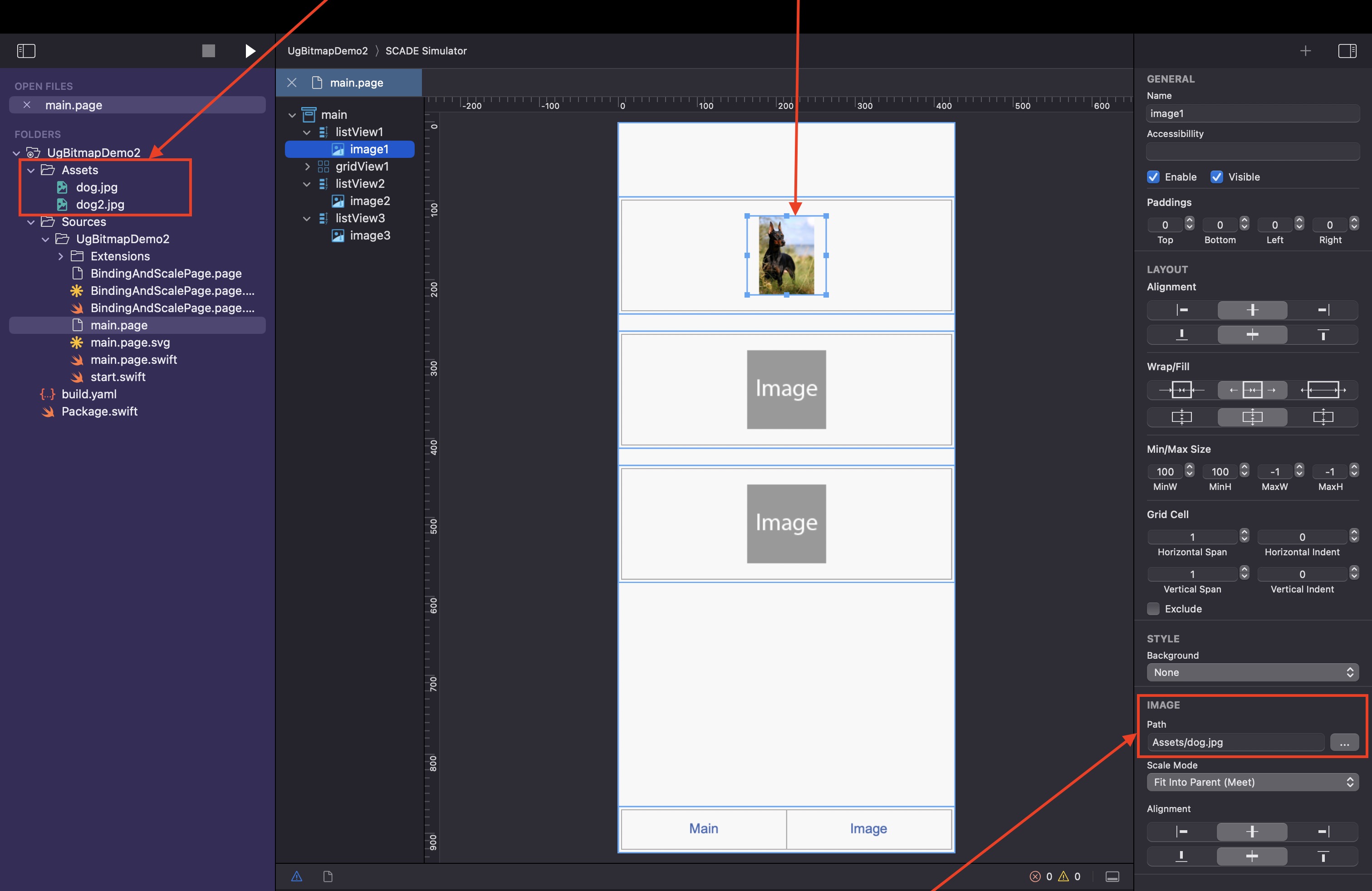
Scale Mode, Alignment and Wrap/Fill
The scale mode describes how the bitmap fits into the current area. You can combine the scale mode and the alignment for best fit.
- Scale mode: none, Fit into Parent (Meet), Fill Parent (slice)
- Alignment (6 different positions)
- Wrap/Fill: Wrap/fill contents in minimal/maximum spaces
Scaling Factor Articles
These articles explain scaling factor in detail. In case its needed
Setting Bitmaps using Code
You always have to make TWO settings
- make sure you specify the path in the url field correctly. It's /<image.png|jpg|svg>
- make sure you set the isContentPriority to false
import ScadeKit
class BindingAndScalePagePageAdapter: SCDLatticePageAdapter {
// page adapter initialization
override func load(_ path: String) {
super.load(path)
self.image2.url = "Assets/dog2.jpg"
self.image2.contentPriority = false
self.main.onClick.append(SCDWidgetsEventHandler{_ in self.navigation!.go(page: "main.page")})
}
}
Setting images using raw data from download
To use images that don't exist on the app yet, we use the SCDNetworkRequest and SCDNetworkResponse objects to download the data:
- set the content field with the raw image data received from the network call
- make sure you set the isContentPriority to true
import ScadeKit
class MainPageAdapter: SCDLatticePageAdapter {
// page adapter initialization
override func load(_ path: String) {
super.load(path)
// adding 2nd page btn
self.itmBinding.onClick{_ in self.navigation!.go(page: "BindingAndScalePage.page")}
// get reference to second bitmap and set bitmap
self.image2.url = "Assets/dog2.jpg"
self.image2.contentPriority = false
// network image to be downloaded
let externalurl = "https://i.pinimg.com/originals/08/2c/ce/082cce3add1418e48ef80208694e8f86.png"
// Create URL
let url = URL(string: externalurl)!
DispatchQueue.main.async {
// Fetch Image Data
if let data = try? Data(contentsOf: url) {
DispatchQueue.main.async {
// Create Image and Update Image Control
self.image3.contentPriority = true
self.image3.content = data
}
}
}
}
}
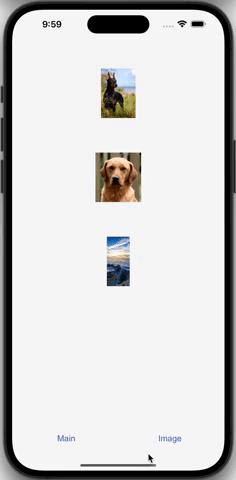
The Image Control Video Tutorial
Updated over 1 year ago