Sliderline control
Slider control to select value on a scale
SourcecodePlease see the UgSliderControlDemo project for the source code created in this chapter. See Installing examples for information on how to download the examples from https://github.com/scadedoc/UgExamples/tree/master/UgSliderControlDemo
Introduction
The SliderLine control is a range control that allows you to set a range. We will rename sliderline to slider control in one of our future releases.
SliderLine Properties and Methods
Variable | Description | Comment |
---|---|---|
onSlide: [SCDWidgetsSlideLineEvent] | Array to append slide events. It has both the oldValue and newValue attributes. |
|
position | Current position within the range | Type Int |
minValue | Minimum range | Type Int. Default 1 |
maxValue | Maximum range | Type Int. Default 100 |
Background Line | It sets the color of the sliderline's inactive part. | Type Color |
Foreground Line | It decides what color the slider line's active area will be. | Type Color |
Thumb | an object that moves horizontally anytime the sliderline control is dragged. | Can be set to either None, Rect, Circle, Elipse or Image |
Bullet | For adjusting the size of the Thumb | Width, Height and Opacity |
touchState | .began |
Working with the SliderLine Control
- Create a new page
- Drag and drop the sliderline control on the page
- Name the control sliderLine
- Use the sliderline control's layout properties to size and position it
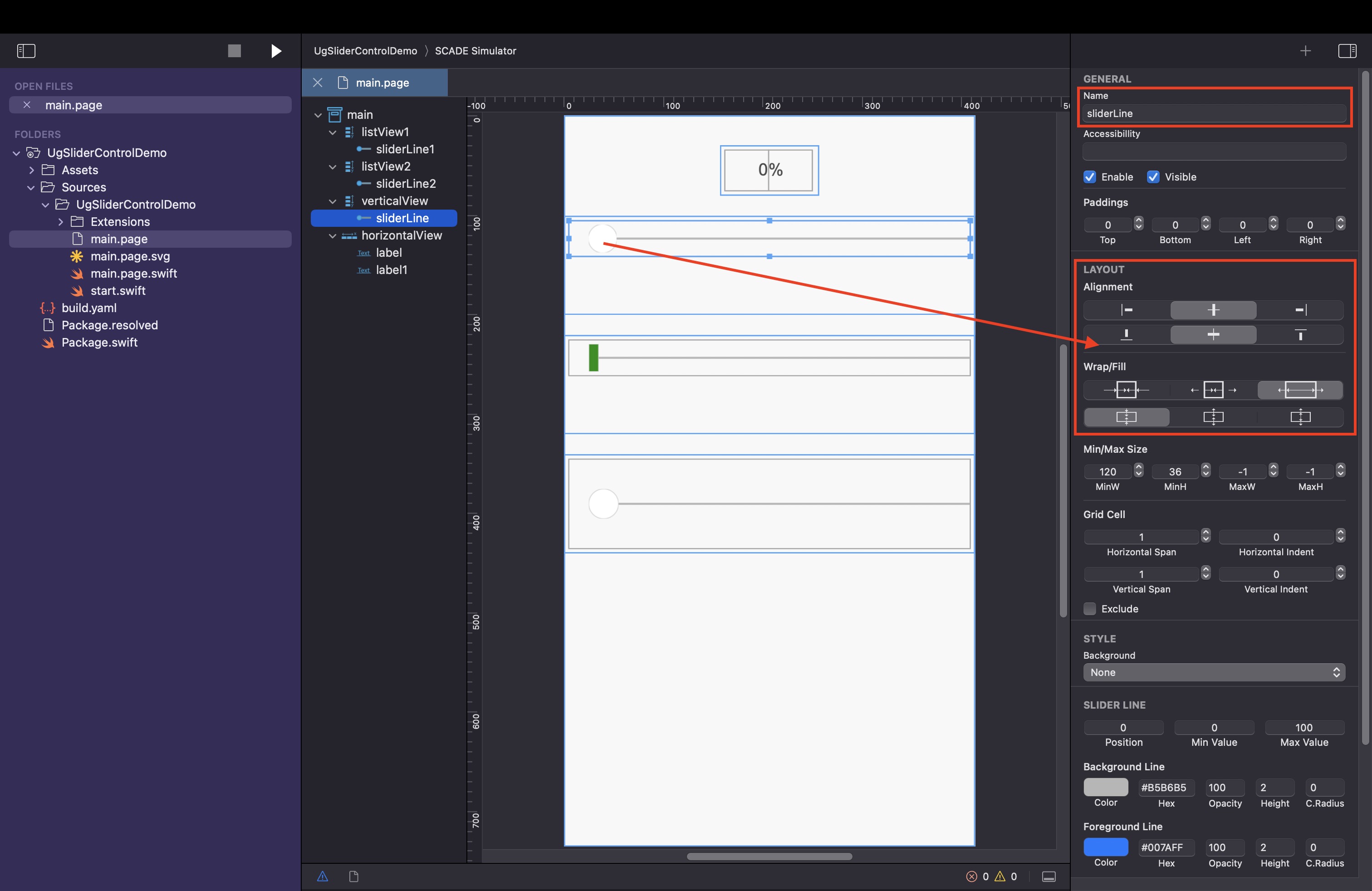
- Use the page editor panel to change the sliderline control properties from their respective default values
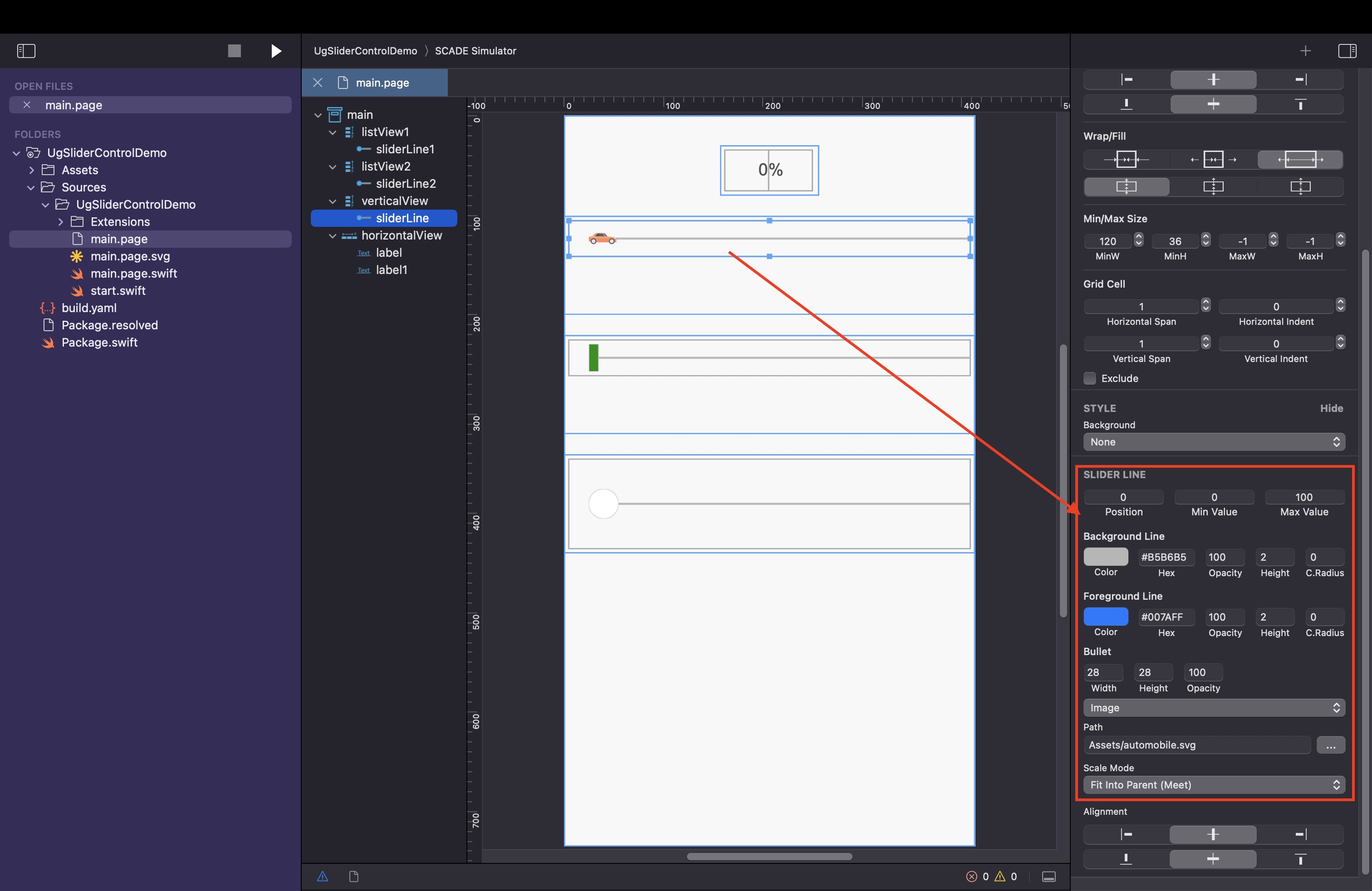
- Set the
label.text
from within the event viaSCDWidgetsSliderLineEventHandler
. Ensure to castev.newValue
to a string before assigning it tolabel.text
for displaying the sliding percentage progress.
import ScadeKit
class MainPageAdapter: SCDLatticePageAdapter {
var percentage: String = "0"
// page adapter initialization
override func load(_ path: String) {
super.load(path)
sliderLine.onSlide.append(SCDWidgetsSliderLineEventHandler { ev in self.onSlide(ev: ev!) })
}
func onSlide(ev: SCDWidgetsSliderLineEvent) {
let oldValue = ev.oldValue
let newValue = ev.newValue
self.percentage = "\(ev.newValue)"
label.text = String(newValue)
print("Old ---> new \(oldValue), \(newValue)")
}
}
This results in
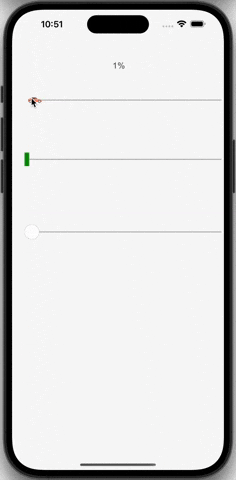
Configuring SliderLine Properties Programmatically
Add the ScadeExtensions repo dependency under the dependencies section of the Package.swift file of your project as it's required for using theScadeUI
library.
.package(name: "ScadeExtensions", url: "https://github.com/scade-platform/ScadeExtensions", .branch("main"))
Currently, using the ScadeUI
dependency is required to programmatically configure the sliderline properties such as the bullet, backgroundLine, and foregroundLine.
import ScadeKit
import ScadeUI
class MainPageAdapter: SCDLatticePageAdapter {
// page adapter initialization
override func load(_ path: String) {
super.load(path)
sliderLine.bullet?.width = 28
sliderLine.bullet?.height = 28
sliderLine.backgroundLine?.height = 2
sliderLine.backgroundLine?.opacity = 100
sliderLine.foregroundLine?.height = 2
sliderLine.foregroundLine?.opacity = 100
}
}
onSlide() Method with the ScadeUI Library
Additionally, with the help of the ScadeUI
library, you can also listen to events via the onSlide()
method without using the SCDWidgetsSliderLineEventHandler
.
import ScadeKit
import ScadeUI
class MainPageAdapter: SCDLatticePageAdapter {
var percentage: String = "0"
// page adapter initialization
override func load(_ path: String) {
super.load(path)
sliderLine.onSlide { event in
print(event.newValue, event.oldValue)
self.percentage = "\(event.newValue)"
self.label.text = String(event.newValue)
}
}
}
Updated 3 months ago