TextField
UITextfield for Android and iOS
Introduction
This chapter describes how to use the textbox control and the keyboard across both the Android and iOS platforms to enter text.
The basic functionalities we provide with the textbox control include:
- showing/hiding the keyboard automatically (and programmatically via code)
- display of the keyboard in overlap mode, where the keyboard panel sits on top of the UI
- display of the keyboard in resize mode, where the UI adjust and resizes when the keyboard is displayed
- you can listen to size changes when the keyboard changes its size
- you can listen to text changes when typing text into the keyboard
- process the event when the send/return key is pressed
- change alphabetic/number type of keyboard
Overview
You can manipulate most of the textbox features using the page editor panel:
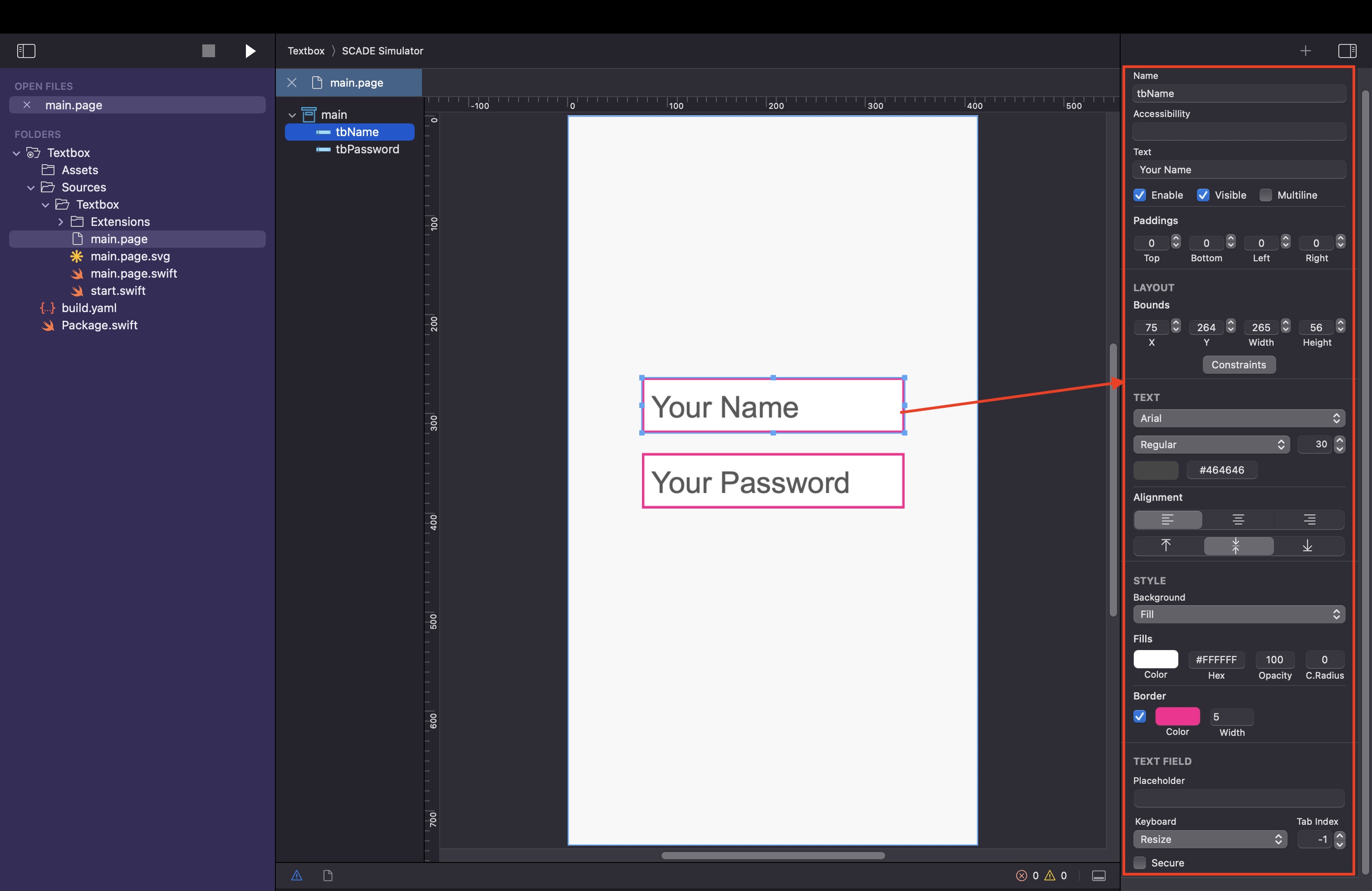
Attribute | Description |
---|---|
Name | The name of the textbox control. SCADE adds a variable with the same name to the page class. |
Accessability | The text to help impaired |
Text | The initial text displayed by the textfield |
Placeholder | The placeholder text displayed by the textfield |
Keyboard | How the keyboard is displayed, options are OnTop or Resize |
Tab Index | Sequence of the textfields |
Secure | Text is masked using iOS and Android masking characters |
Keyboard type | It can be set to alphabetic or number. Currently not possible through page builder. Please use attribute. |
Textfield Events
We can capture the following events
- onEditFinished - when a user clicks the return or tab key, or when they leave the textbox control
- onTextChanged - when the value of the textfield changes
The code snippet below shows an example each on how to make use of the two methods:
import ScadeKit
class MainPageAdapter: SCDLatticePageAdapter {
// page adapter initialization
override func load(_ path: String) {
super.load(path)
// define the logic onEditFinish
self.tbName.onEditFinish.append(SCDWidgetsEditFinishEventHandler{
ev in print("Finished Editing. New value:" + ev!.newValue)
})
// define the logic onTextChange
self.tbName.onTextChange.append(SCDWidgetsTextChangeEventHandler{
ev in print("Value has changed to : " + ev!.newValue )
})
}
}
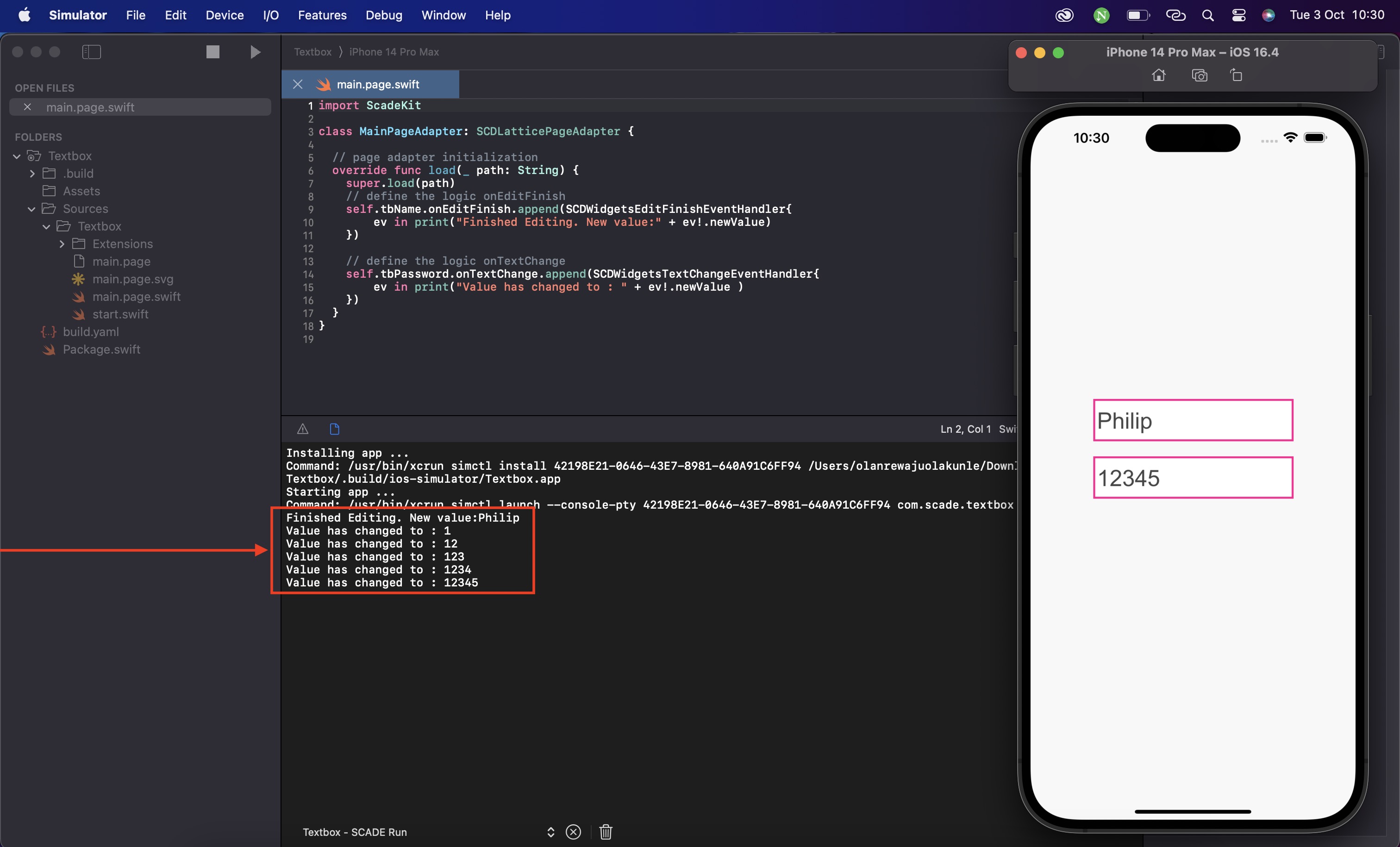
Building an App that uses Resizing / Shifting
Many apps require that controls shift and/or resize in case the keyboard is displayed. For instance, imagine a form that you fill out, and the textfield to fill out is overlaid by the keyboard control. In that case, you want to use the Resize mode to shift up the control to make it possible for the user to see the textfield the user is entering the text in.
By default, the keyboard is set to Resize mode but we can always use the page editor panel to change it to the OnTop mode if that is what our app require.
Click on the preferred textbox and stroll to the keyboard section to make this happen:
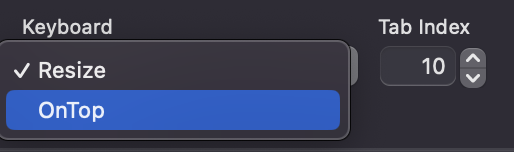
Alternatively, we can programmatically change the keyboard mode to OnTop type via the keyboard attribute:
class MainPageAdapter: SCDLatticePageAdapter {
// page adapter initialization
override func load(_ path: String) {
super.load(path)
let textbox1 = self.page!.getWidgetByName("textbox1") as! SCDWidgetsTextbox
textbox1.keyboard = .onTop
}
}
Switch to numeric keyboard
By default the textField is displayed with an alphabetical keyboard type. But we can change the type of the keyboard by setting the keyboardType attribute to the numerical keyboard type:
class MainPageAdapter: SCDLatticePageAdapter {
// page adapter initialization
override func load(_ path: String) {
super.load(path)
let textbox1 = self.page!.getWidgetByName("tbPassword") as! SCDWidgetsTextbox
textbox1.keyboardType = .number
}
}
This results in
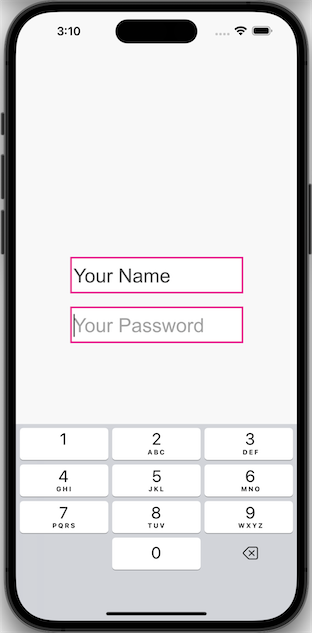
Hide the keyboard
The keyboard is displayed automatically when a user enters texts into a textbox. Sometimes there is a need to use code to hide the keyboard. This is done using the .hideKeyboard()
method
// hide the keyboard on Android and iOS
SCDRuntime.getSystem().hideKeyboard()
Updated 10 months ago