WebView control
Version 2.0 (December 2021)
Introduction
Many mobile apps need a webview. We wrapped the native webView control from iOS (WKWebView) and Android (android.webkit.WebView), respectively, and accessed its functionality using one common interface and API.
Functionalities include:
- positioning a webView control anywhere within the app page
- loading a webpage
- receiving events when the page was loaded
- preventing pages on loading
- injecting and evaluating Javascript
What a Minimal WebView App Looks Like
- Create a new mobile app project
- Drag and drop the webView onto the page
- Add a button
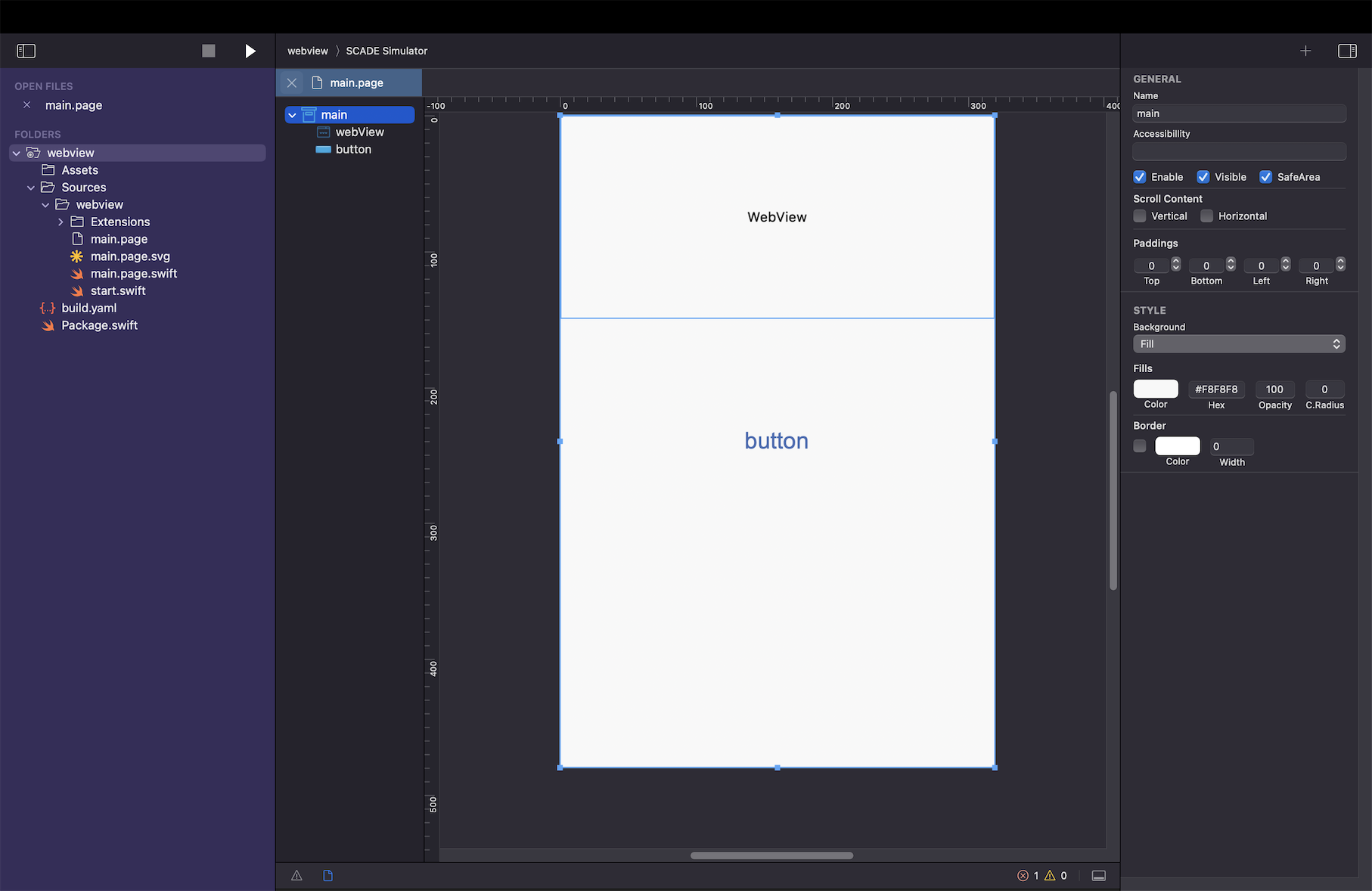
- Add this minimal code below to reference the webView control in the main.page.swift file and load the UIKit page:
import ScadeKit
class MainPageAdapter: SCDLatticePageAdapter {
let webpage = "https://developer.apple.com/reference/webkit/wkwebview"
// page adapter initialization
override func load(_ path: String) {
super.load(path)
// Add event to load page
button.onClick{
_ in self.webView.load(self.webpage)
}
// Add event when page loaded
webView.onLoaded.append(SCDWidgetsLoadEventHandler{
(ev:SCDWidgetsLoadEvent?) in print("Page Loaded: \(ev!.url)")})
// Add event when page failed to load
webView.onLoadFailed.append(SCDWidgetsLoadFailedEventHandler{
(ev:SCDWidgetsLoadFailedEvent?) in print("Page failed to load \(ev?.message)")})
}
}
Events on successful and failed load of pages
The _SCDWidgetsLoadEvent_
indicates the successful load of the page, and the url indicates the page that we loaded successfully. Similarly, the _SCDWidgetsLoadFailedEvent_
is triggered when a failure occurs.
Controlling if a page should be loaded
In some cases, we may want to prevent the loading of some pages based on the URL. This can be done with the _SCDWidgetsShouldLoadEventHandler_.
Return FALSE to indicate that the URL should not be loaded:
// Add handler to control loading of page
webView.onShouldLoad.append(SCDWidgetsShouldLoadEventHandler{
(ev:SCDWidgetsLoadEvent?) in
let rtn = !ev!.url.contains("loadhtmlstring")
print("will load \(ev!.url) : \(rtn)")
return rtn
})
Injecting Javascript
The eval
function allows you to pass JavaScript and get the result. In this example, we print the value of the <title>
tag:
func getTitle() {
let javascript = "var x=document.getElementsByTagName(\"title\")[0].text.toString();x"
let processResult = SCDWidgetsWebViewEvalHandler { result in print(result)}
let handleException = SCDWidgetsWebViewEvalHandler { error in print(error)}
// inject javascript
webView.eval(javascript, onSuccess: processResult, onError: handleException)
}
The WebView Control Video Tutorial
Updated over 1 year ago