System Guide
Requires Version 0.9.9.9 or higher
This guide explains how to implement system specific functions
- Capture the events when an application enters the background or foreground
- Explains the implementation of deep linking
Examples
Please see the UgSystemDemo project for the source code created in this chapter. See Installing examples for information on how to download the examples.
Background and Foreground events
OS | Functional similar to |
---|---|
iOS | applicationWillResignActive willResignActiveNotification appDidBecomeActive |
Android | onResume() onRestart() |
You need to override the onEnterForeground and onEnterBackground functions to capture the event when an applications enters the Foreground and Background
import ScadeKit
class UgSystemDemo: SCDApplication {
let window = SCDLatticeWindow()
let mainAdapter = MainPageAdapter()
override func onFinishLaunching() {
mainAdapter.load("main.page")
mainAdapter.show(window)
}
// Override method to capture background enter event
override func onEnterBackground() {
print("onEnterBackground")
}
// Override method to capture foreground enter event
override func onEnterForeground() {
print("onEnterForeground")
}
}
SCADE API uses the UIApplicationDelegate api on Apple, and the Activity class on Android to capture these system events.
Deep Linking
SCADE provides access to iOS and Android deep linking functionality using one API offered through the SCADE SDK.
You can create an app handler that reacts to any Deep Link call and provides the necessary data about the call.
Here some intro document references for optional reading, the below tutorial covers all the basics.
- Android https://developer.android.com/training/app-links/deep-linking
- iOS https://medium.com/@stasost/ios-how-to-open-deep-links-notifications-and-shortcuts-253fb38e1696
Getting started
A deep link consists of a project-uri followed by the rest of the link
You can use any string you want as project-uri BUT the string needs to be unique across all apps. We use ScadeDeepLinking as the project-uri.
Therefore the link to call any functionality on the app would be:
Add Deep Link information to build file
As a first step, you need to add information to the build file so that Android and iOS know about the fact that you want to use Deep Linking and register that information with Android and iOS.
Please add the following segment to the 'ios:' part of your build file
plist:
- key: CFBundleURLTypes
type: list
values:
- type: dict
values:
- key: CFBundleURLName
type: string
value: project-id
- key: CFBundleURLSchemes
type: list
values:
- type: string
value: project-uri
And the following segment to the 'android:' part of your build file
intent-filters:
- action: android.intent.action.VIEW
scheme: project-uri
categories: [android.intent.category.DEFAULT, android.intent.category.BROWSABLE]
Within the YAML, you need to make 3 modifications
- For iOS, set the value for CFBundleURLName
- For iOS, set the project-uri as the value for the CFBundleURLSchemes items array
- For Android, set the project-uri as the value set the scheme field
We marked the values you have to change in your app here:
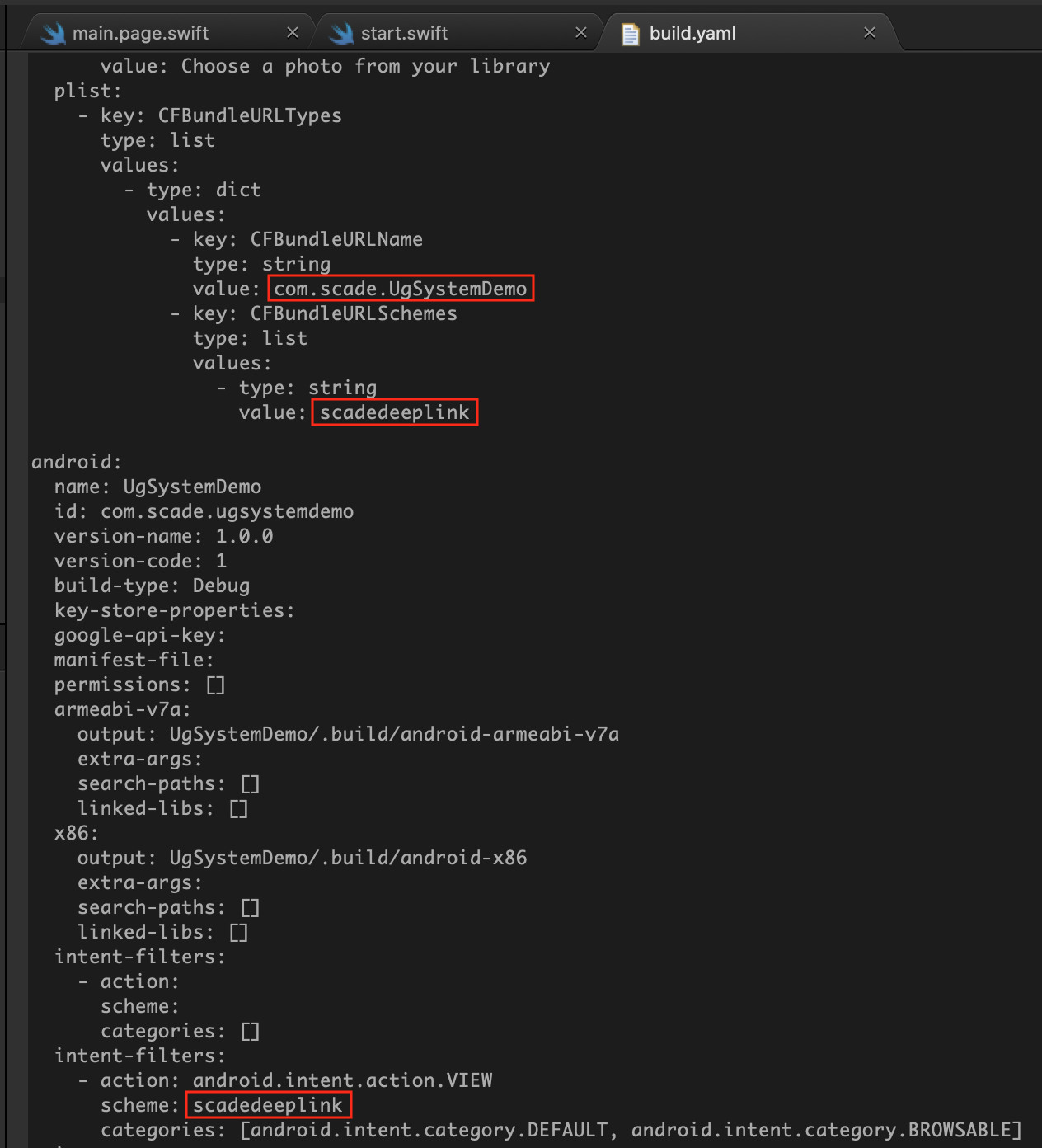
Add code to handle incoming Deep Link calls
As a second step, you need to implement the code that is called when the deep link is invoked from outside. Goto the start.swift file and override the onOpen method:
Whenever the deep link is invoked, you receive the URL in the url parameter. The below code demos extracting the information from the URL and in this example informs the main window if the link contains a matching parameter name.
override func onOpen(with url: String) {
if let ucs = URLComponents(string:url) {
// The host value
print(String(ucs.host!))
// This is how to iterate the queryItems
for qp in ucs.queryItems! {
print(qp.name,String(qp.value!))
}
// lets search for a query parameter named msg and if it exists, inform window
if let msg = ucs.queryItems!.first(where: { $0.name == "msg" } ) {
mainAdapter.newDeepLinkMsg(msg:msg.value!)
}
}
}
Compile and test
Testing your deep link can only happen in iOS or Android simulator or app:
- You require Android API 23 or higher
Testing on iOS
- run the app on iOS simulator.
- Goto Safari and type scadedeeplinking://try?msg=ScadeRocks
- our app will start up, and the message ScadeRocks will appear on the main screen
Testing on Android
Android doesn't allow direct deep link invocation via the browser. One way of testing deep links is using the adb:
- run the app on Android simulator with API 23 or higher
- Run the adb am command to invoke a deep link
./adb shell am start -a android.intent.action.VIEW -d "scadedeeplink://try?msg=ScadeRocks" com.scade.ugsystemdemo
Deep Link testing on Android - ADB AM command for calling a deep link
./adb shell am start -a android.intent.action.VIEW -d "scadedeeplink://try?msg=ScadeRocks" com.scade.ugsystemdemo
Its important that com.scade.ugsystemdemo MUST be lowercase
Gotchas
Here some hints of possible mistakes you might have made if things aren't working
Check .build file
You might have a typo in the .build file. In case of a typo, the compiler information for cmake is not created correctly.
- Check the .build file for typos
- Check the CMakeList.text file. Make sure they contain the correct entries. These are autogenerated after compiling to iOS/Android. The highlighted section contains the entries needed for deep linking:

Updated 7 months ago